#include <string.h>
#include <stdint.h>
#include <sstream>
#include <vector>
#include <string>
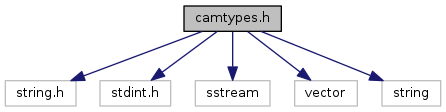
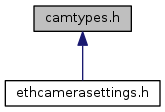
Go to the source code of this file.
Classes | |
struct | CrossControl::ImagerRegister |
Definition of an address/value pair of imager register. More... | |
struct | CrossControl::TimeStamp |
Definition of a timestamp. More... | |
struct | CrossControl::VideoContent |
Defines the format of a video content notification package. More... | |
struct | CrossControl::CamStatus |
Defines the camera status information. More... | |
struct | CrossControl::ControlStatus |
Definition of a camera control mode register. More... | |
struct | CrossControl::SignedControl |
Definition of a signed camera control. More... | |
struct | CrossControl::UnsignedControl |
Definition of a unsigned camera control. More... | |
struct | CrossControl::CombinedControl |
Definition of a combined camera control. More... | |
struct | CrossControl::PixelPosition |
Definition of the position of a single pixel relative to a window. (0,0) Is the top left corner. The effects of the optical system (e.g. lens) are included. More... | |
struct | CrossControl::Rectangle |
Definition of a rectangular area using two pixels coordinates. More... | |
struct | CrossControl::PixelMap |
Defines a group of independent pixels. This structure is used, for instance, to report the position of defect pixel in the imager. More... | |
struct | CrossControl::ImageDimension |
Definition of the image dimension, in pixels. More... | |
struct | CrossControl::ImagerCharacteristic |
Defines the characteristics of the camera. More... | |
struct | CrossControl::IntrinsicCamParam |
The intrinsic camera parameters determine the projection from 3-D camera coordinates to 2-D pixel coordinates. The intrinsic parameters of the camera can be read out by application software and can be used for back-projection form a 2-D image position to a line in 3-D space. More... | |
struct | CrossControl::ExtrinsicCamParam |
The extrinsic camera parameters determine the mapping from 3-D vehicle coordinatesto 3-D camera coordinates. The extrinsic parameters of the camera can be written and read out by application software and can be used, in conjunction with the intrinsic camera parameters, for backprojection form a 2-D image position to a line in 3-D space that is defined in terms of the vehiclecoordinate system. More... | |
struct | CrossControl::Datasheet |
Defines the characteristics of the camera. More... | |
struct | CrossControl::HistogramFormat |
Defines the histogram format used for a certain region of interest. More... | |
struct | CrossControl::VideoFormat |
Defines the parameter of the video format associated to certain region of interest link text ... . More... | |
struct | CrossControl::RegionOfInterest |
Defines the region of interest. The ROIs (regions of interest) are used to acquire data from the camera image using subscribe/notification mechanism. The data can be the image content of the region itself or a histogram of the region. ROIs are interpreted as an array of structures and referenced by its index (roiIndex). More... | |
struct | CrossControl::SomeIPHeader |
The sSomeIPHeader struct A struct containg the SOME/IP header data. More... | |
struct | CrossControl::SDEntryA |
The sSDEntryA struct A struct containing the Service Discovery Entry type A. More... | |
struct | CrossControl::IPv4Option |
The sIPv4Option struct Describes the IPv4EndpointOption. More... | |
Detailed Description
Contains the datatypes, structures and enums that are used the API
Enumeration Type Documentation
enum CrossControl::ColorSpace : uint8_t |
The ColorSpace enum. Vendor codes starting at 128 can be used for specific codec profiles.
enum CrossControl::ControlMode : uint8_t |
enum CrossControl::ControlSupportMode : uint8_t |
Defines the valid values for the supported modes of operation of camera controls.
enum CrossControl::EntryType : uint8_t |
The EntryType enum Describes what type of intent the Service Discovery Message has.
Returncodes and error messages. Can be sent from either the camera or the API.
Enumerator | |
---|---|
E_OK |
No error occurred |
E_NOT_OK |
An unspecified error occurred |
E_UNKNOWN_SERVICE |
The requested Service ID is unkown |
E_UNKNOWN_METHOD |
The requested Method ID is unkown. Service ID is know |
E_NOT_READY |
Service ID and Method ID are known. Application not running |
E_NOT_REACHABLE |
System running the service is not reachable |
E_TIMEOUT |
A timeout occurred |
E_WRONG_PROTOCOL_VERSION |
Version of SOME/IP protocol not supported |
E_WRONG_INTERFACE_VERSION |
Interface version mismatch |
E_MALFORMED_MESSAGE |
Deserialization error (i.e. length or type incorrect) |
E_LOCKED_BY_FOREIGN_INSTANCE |
Camera service is already locked by another client |
E_LOCK_EXPIRED |
The camera lock has expired |
E_NOT_LOCKED |
Camera is not locked |
E_INVALID_PS_ENTRY |
The requested PSE ID is unknown |
E_INVALID_PS_OPERATION |
The requested PSE operation is not allowed, e.g. store on a RO PSE |
E_INVALID_PS_DATA |
The PSE contains a CRC16 error |
E_NO_MORE_SPACE |
No more space available to store the PSE |
E_INVALID_ROI_INDEX |
The requested ROI is out of range |
E_INVALID_ROI_NUMBER |
The requested number of ROIs is out of range, defined by Datasheet.numRegionsOfInterest |
E_INVALID_VIDEO_FORMAT |
Invalid value in video format |
E_INVALID_HISTOGRAM_FORMAT |
Invalid value in histogram format |
E_INVALID_CONTROL_INDEX |
The requested camControlIndex is out of range or not supported by the camera |
E_INVALID_CONTROL_MODE |
The requested control mode is not supported by the camera |
E_INVALID_CONTROL_VALUE |
The requested control value is out of the range, defined in CamControl |
E_INVALID_REGISTER_ADDRESS |
The register address is not supported by the imager |
E_INVALID_REGISTER_VALUE |
The value for the given register address is not supported by the imager |
E_INVALID_REGISTER_OPERATION |
The requested operation (read or write) for the given register address is not supported by the imager |
E_SOCKET_ERR |
There was an unspecified socket error |
E_SOCKET_ERR_SEND |
There was an error on writing to the socket |
E_NO_RESPONSE |
There was no matching message in the socket |
enum CrossControl::L4Protocol : uint8_t |
enum CrossControl::MessageType : uint8_t |
The MessageType enum Describes what kind of intent a SOME/IP message has.
enum CrossControl::MethodID : uint16_t |
The MethodID enum Describes the different methods.
enum CrossControl::OptionType : uint8_t |
The OptionType enum Describes different options sent by Service Discovery messages.
enum CrossControl::ServiceID : uint16_t |
enum CrossControl::VideoCompression : uint8_t |